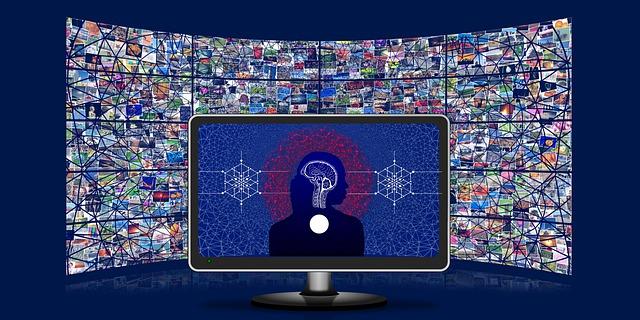
5 Algorithms Every Developer Should Know In 2023
21 March 2023Understanding Angular Unit Testing: The Car Profiles Case
Writing unit tests can often seem a complex task for many Angular developers. This complexity mainly stems from either a lack of familiarity or uncertainty about the proper approach to take. This article aims to introduce a straightforward method that employs a practical example to simplify the process of writing these tests. To this end, we will rely on the Jasmine/Karma testing duo, natively integrated when creating an Angular project.
Indeed, Jasmine is a framework that allows us to quickly write our unit tests, while Karma is a server that enables us to run our tests, providing a live feedback loop of what’s happening in our browser.
Car Name Display Code
Let’s assume we want to display the name of a car as soon as we click a start button. For this purpose, we’ll use a component named CarProfileComponent.
In other words, this component is specifically created to display the name of a car (carName) as soon as a user clicks a (Start) button. Here is the code snippet dedicated to this display:
import { Component, Input, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-car-profile',
template: `
<div>
<p>{{ carName }}</p>
<button (click)="emitStartEvent()">Start</button>
</div>
`,
})
export class CarProfileComponent {
@Input() carName ;
@Output() startEvent = new EventEmitter<string>();
emitStartEvent(): void {
this.startEvent.emit(this.carName);
}
}
In this example, we find that our CarProfileComponent includes a `carName` property as an input and a `startEvent` as an output.
Next, we’ll integrate this reusable component and call it from a parent component as follows:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<div>
<app-car-profile [carName]="'Toyota'" (startEvent)="handleStartEvent($event)"></app-car-profile>
</div>
`,
})
export class AppComponent {
handleStartEvent(name: string): void {
console.log(`The car ${name} start !`);
}
}
Integrating Unit Tests
Typically, when creating an Angular component, a unit testing file with the extension `.spec.ts‘ is generated; this is where the unit tests related to our component should be stored.
If we examine the `Car-profile.component.spec.ts` file, referring to our CarProfileComponent, we will find the following baseline code:
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { CarProfileComponent } from './car-profile.component';
describe('CarProfileComponent', () => {
let component: CarProfileComponent;
let fixture: ComponentFixture<CarProfileComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [CarProfileComponent],
}).compileComponents();
fixture = TestBed.createComponent(CarProfileComponent);
component = fixture.componentInstance;
component.carName = 'Toyota';
fixture.detectChanges();
});
it('should be created', () => {
expect(component).toBeTruthy();
});
it('should display carName', () => {
const elementMessage = fixture.nativeElement.querySelector('p');
expect(elementMessage.textContent).toContain('Toyota');
});
it('should emit startEvent', () => {
spyOn(component.startEvent, 'emit');
component.emitStartEvent();
expect(component.startEvent.emit).toHaveBeenCalledWith('Toyota');
});
});
Running these unit tests with the appropriate command (e.g., `ng test`) allows observation of the results in the console.
Upon executing this code, an instance of the CarProfileComponent to be tested is created, a call to the `emitStartEvent method is simulated, and the emission of the `startEvent` with the value ‘Toyota‘ is verified.
Ultimately, as demonstrated through this example, writing unit tests is not as daunting a task as it might seem.
We hope that our unit tests on an Angular application component, utilising properties and events with the example of car profiles, have helped to demystify them.
Unit testing remains an important part of the software development process. Thus, we trust this article will serve as a good introduction to inspire more confidence and reduce hesitations related to this practice 😉 .